Writing your first bot¶

An example of what you can build using Pyrubrum (see Calendar Bot).¶
Introduction to the forest data structure¶
You may have noticed it actually isn’t a tree, as the main menu is within a set as well. The fact is that Pyrubrum does actually work with forest data structures, which are just a collection of multiple trees. That means you can define multiple top-level menus!
Let’s say we want to create two top-level menus: we’ve already defined one of them before and we now decide to create a new one for contacting you using the command /contact
.
Then the structure has changed to this:
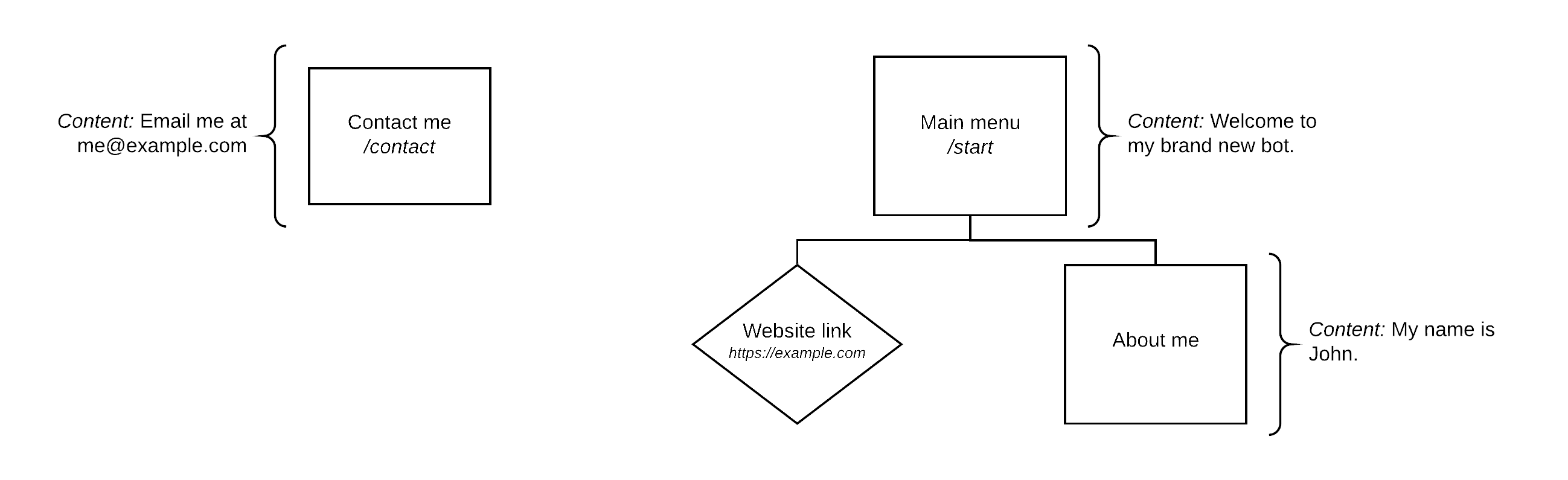
Which translates to:
from pyrubrum import LinkMenu, Menu
...
{
Menu("Main menu", "start", "Welcome to my brand new bot."): [
LinkMenu("Website link", "website_link", "https://example.com"),
Menu("About me", "about_me",
"My name is John."),
],
Menu("Contact me", "contact", "Email me at me@example.com"): [],
}
Congratulations! You’ve just written your first forest!
Submitting a forest to an handler¶
The forest structure we’ve just built up does not do anything by itself. As a result of this, we need something which is able to handle the messages that our bot is going to receive and redirects them to our menus. And what can handle something better than an handler itself?
Parameterized vs non-parameterized handler¶
The are two types of handler: the parameterized one (i.e. ParameterizedHandler
) and the other one which is not (i.e. Handler
).
Using the first one, you can submit parameters to the bot, store them, add and update information about a user and do much more.
Being our bot a constant bot, we are fine with using an handler that does not parameterization.
Setting an handler¶
Since Pyrubrum already makes it simple, we just have to pass the forest as argument to our handler. Our example is then going to look like this:
from pyrubrum import Handler, LinkMenu, Menu, transform
...
handler = Handler(transform({
Menu("Main menu", "start", "Welcome to my brand new bot."): [
LinkMenu("Website link", "website_link", "https://example.com"),
Menu("About me", "about_me",
"My name is John."),
],
Menu("Contact me", "contact", "Email me at me@example.com"): [],
}))
And now we’re done with the programming part related to Pyrubrum.
Creating a bot¶
In order to access Telegram APIs, we need to have an authorization token that links to the bot you want to make use of, which you can retrieve by talking to BotFather. You will also need to create your own API credentials (see https://my.telegram.org/apps).
As soon as you get your credentials, we can start by importing the Pyrogram library and creating a pyrogram.Client
instance:
from pyrogram import Client
from pyrubrum import Handler, LinkMenu, Menu, transform
bot = Client("ExampleBot",
api_hash="<YOUR_API_HASH_GOES_HERE>",
api_id="<YOUR_API_ID_GOES_HERE>",
bot_token="<YOUR_BOT_TOKEN_GOES_HERE>"
)
handler = Handler(transform({
Menu("Main menu", "start", "Welcome to my brand new bot."): [
LinkMenu("Website link", "website_link", "https://example.com"),
Menu("About me", "about_me",
"My name is John."),
],
Menu("Contact me", "contact", "Email me at me@example.com"): [],
}))
We did it! We’re now ready for the final part of this tutorial.
Setting up & running a bot¶
Given an handler, we just have to use the method setup
and pass our bot instance as argument. Finally, to run it, we will have to call the run
method of our bot.
It is then built in this way:
from pyrogram import Client
from pyrubrum import Handler, LinkMenu, Menu, transform
bot = Client("ExampleBot",
api_hash="<YOUR_API_HASH_GOES_HERE>",
api_id="<YOUR_API_ID_GOES_HERE>",
bot_token="<YOUR_BOT_TOKEN_GOES_HERE>"
)
handler = Handler(transform({
Menu("Main menu", "start", "Welcome to my brand new bot."): [
LinkMenu("Website link", "website_link", "https://example.com"),
Menu("About me", "about_me",
"My name is John."),
],
Menu("Contact me", "contact", "Email me at me@example.com"): [],
}))
handler.setup(bot)
bot.run()
Hooray! We’ve just finished creating our first bot. You’re now ready to explore the other available examples. Check the API documentation as well to deeply understand how Pyrubrum works and to get to know other features that weren’t featured in this brief tutorial.
Keep it up!